Introduction
In the realm of web development, databases serve as the bedrock of our applications. Among the various database systems, PostgreSQL stands out as a reliable and feature-rich choice. In this guide, we will explore the process of connecting PostgreSQL with Spring Boot, a popular framework for Java-based applications. By the end of this journey, you’ll be well-equipped to create robust, database-driven applications.
Prerequisites
Before we begin, ensure you have the following essentials:
- Java (preferably JDK 17) installed.
- Set up a Spring Boot project; you can use Spring Initializer for this purpose.
- PostgreSQL installed.
- A database created; you can use PgAdmin, PostgreSQL’s GUI interface.
- An IDE to run the application; preferably IntelliJ IDEA.
Steps to Establish a Connection:
Step 1: Adding PostgreSQL Dependency
Our first step towards PostgreSQL-Spring Boot integration involves adding the PostgreSQL JDBC driver to your project. Open your project’s pom.xml file and introduce this dependency:
<dependency>
<groupId>org.postgresql</groupId>
<artifactId>postgresql</artifactId>
<version>42.6.0</version> <!-- Always use the latest version -->
</dependency>
This dependency serves as the crucial link enabling communication between your Spring Boot application and the PostgreSQL database.
Step 2: Configuration in Application Properties
To establish database connections, Spring Boot relies on configuration properties. In your application.properties or application.yml file, furnish the necessary PostgreSQL connection properties:
application.properties:
spring.datasource.url=jdbc:postgresql://localhost:5432/mydatabase
spring.datasource.username=db_username
spring.datasource.password=db_password
spring.datasource.driver-class-name=org.postgresql.Driver
application.yml:
spring:
datasource:
url: jdbc:postgresql://localhost:5432/mydatabase
username: db_username
password: db_password
driver-class-name: org.postgresql.Driver
Replace mydatabase with your PostgreSQL database name.
Set db_username and db_password to your PostgreSQL credentials.
Step 3: Creating a Data Entity
In Spring Boot applications, we often use the Java Persistence API (JPA) to interact with databases. Create an entity class that represents a table in your PostgreSQL database. Here’s an example:
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
private String email;
// Getter and setter methods
}
This entity class maps directly to a “users” table in your PostgreSQL database.
Step 4: Developing a Repository
Next, design a repository interface that extends JpaRepository. This interface facilitates CRUD (Create, Read, Update, Delete) operations on your entity. For our “User” entity, it might look like this:
public interface UserRepository extends JpaRepository<User, Long> {
// Define custom query methods if needed
}
Spring Data JPA automatically generates the required database queries based on method names.
Step 5: Building a Service
Create a service class that interacts with the repository, providing the application’s business logic. Here’s a straightforward example:
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public List<User> getAllUsers() {
return userRepository.findAll();
}
public User createUser(User user) {
return userRepository.save(user);
}
// Include additional service methods as required
}
Step 6: Establishing REST Endpoints
To make your service methods accessible via HTTP requests, employ Spring Web to design a controller. Map HTTP requests to service methods like this:
@RestController
@RequestMapping("/api/users")
public class UserController {
@Autowired
private UserService userService;
@GetMapping
public List<User> getAllUsers() {
return userService.getAllUsers();
}
@PostMapping
public User createUser(@RequestBody User user) {
return userService.createUser(user);
}
// Add more endpoints as necessary
}
Step 7: Running Your Application
With all components in place, run your Spring Boot application. It should seamlessly connect to your PostgreSQL database using the provided configuration.
Conclusion
Integrating PostgreSQL with Spring Boot is a crucial step toward creating powerful, data-driven applications. This guide has walked you through adding PostgreSQL as a data source, configuring the connection, defining entities, repositories, services, and REST endpoints. From this foundation, you can expand to construct influential Spring Boot applications that leverage PostgreSQL’s capabilities for data storage and retrieval.
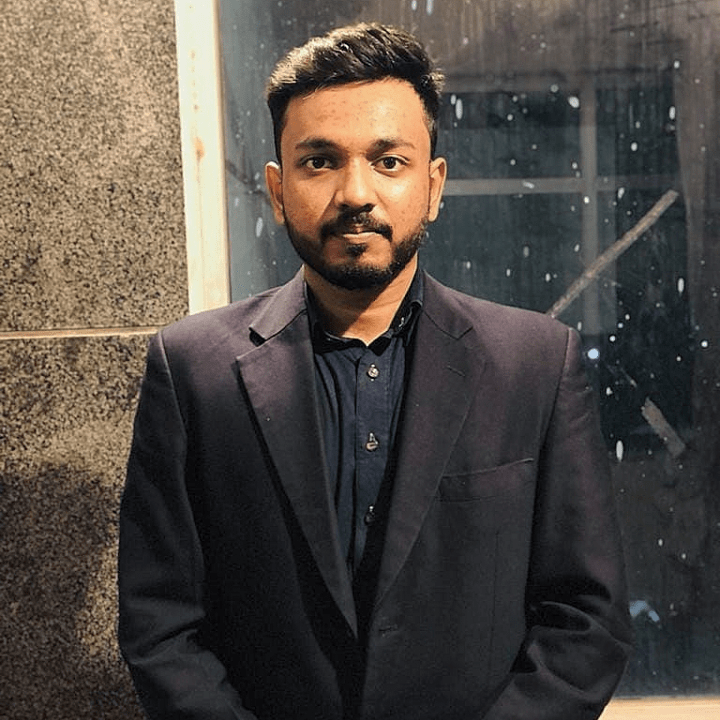